Python has become a powerhouse in the world of programming, and mastering the art of writing Python code is crucial for modern developers. Whether you’re crafting a simple script or building complex software written in Python, understanding the latest techniques and best practices can significantly boost your productivity and code quality. You’ll find that staying up-to-date with modern Python features is not just beneficial—it’s essential to stay competitive in today’s fast-paced tech landscape.
In this article, you’ll discover key strategies to elevate your Python programming skills. We’ll explore how type hinting can improve your code’s reliability, delve into effective project management and dependency handling, and uncover the power of new Python syntax. By the end, you’ll have a solid grasp on writing modern Python programs that are not only efficient but also maintainable and scalable. Get ready to transform your approach to writing Python and take your development skills to the next level.
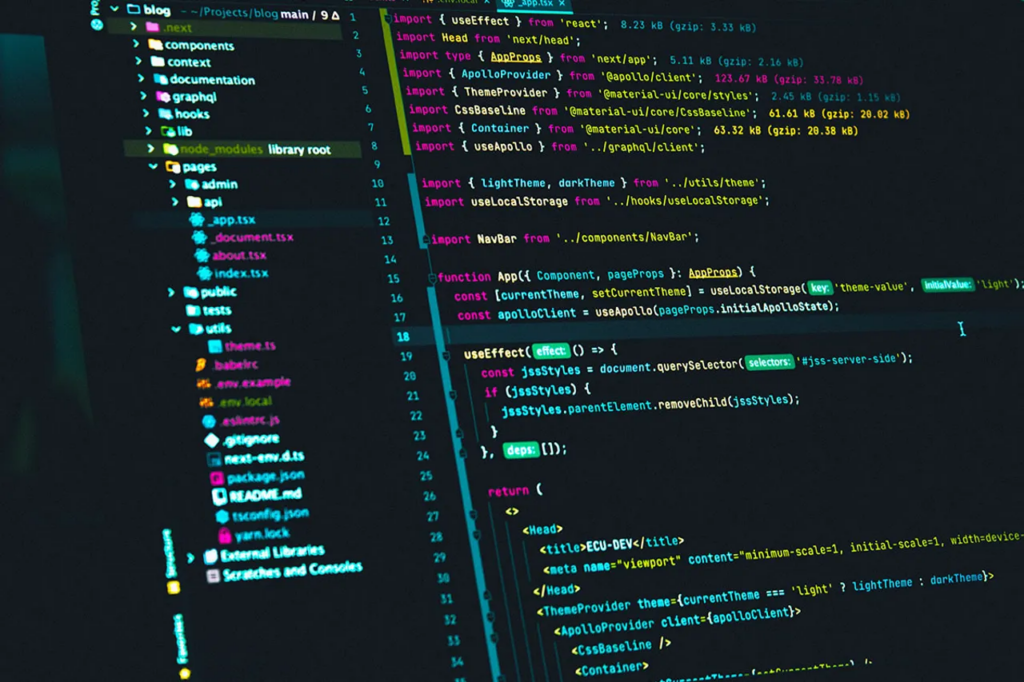
Elevating Code Quality with Type Hinting
Type hinting, introduced in Python 3.5, has become a powerful tool to enhance code quality and reliability. By providing a way to specify expected types for variables, function parameters, and return values, type hints offer a map for developers to understand data flow and prevent potential errors .
Introduction to Type Hints
Type hints allow you to annotate your code with expected types, making it easier to catch bugs before runtime. For instance, you can specify function parameters and return types like this:
def greet(name: str) -> str:
return f"Hello, {name}"
This simple annotation indicates that the name parameter should be a string, and the function will return a string . While Python remains dynamically typed, these hints serve as a guide for developers and tools alike.
Advanced Type Hinting Techniques
As you delve deeper into type hinting, you’ll encounter more complex scenarios. The typing module provides several advanced features to handle these cases:
- Generic Types: Use TypeVar to create generic functions or classes that work with different types .
- Union Types: Employ Union to specify that a variable can be one of several types .
- Optional Types: Utilize Optional for values that might be None .
- Callable Types: Use Callable to hint at function types with specific input and output types .
Here’s an example of a more complex type hint:
from typing import List, Union
def process_data(items: List[Union[int, str]]) -> None:
for item in items:
print(item)
This function accepts a list containing either integers or strings .
Integrating Type Hints with IDEs and Linters
One of the most significant benefits of type hinting is its integration with development tools. IDEs and linters can leverage these hints to provide better code completion, error checking, and refactoring suggestions .
Tools like mypy and pyright perform static type checking based on your type hints, alerting you to potential errors before runtime . To get the most out of type hinting, consider these steps:
- Use a type-aware IDE like PyCharm or Visual Studio Code.
- Integrate a static type checker into your development workflow.
- Set up pre-commit hooks to enforce type checking in your projects .
By incorporating these practices, you’ll find that type hints not only improve code quality but also enhance the overall development experience. They make your code more self-documenting, easier to understand, and less prone to errors .
Optimizing Project Management and Dependency Handling
Virtual Environments: Beyond venv
Virtual environments are crucial for Python development, providing lightweight and isolated spaces for your projects. They help you avoid system pollution, sidestep dependency conflicts, and minimize reproducibility issues . While the built-in venv module is commonly used, there are alternatives worth exploring.
One such alternative is microvenv, which offers a simpler approach by omitting activation scripts. This can be beneficial if you’re working with tools like VS Code that are moving towards implicit activation . Remember, you don’t always need to activate a virtual environment to use it – you can simply use the absolute path to the Python interpreter in your virtual environment when running your script.
Modern Dependency Management Tools
The landscape of Python packaging has evolved significantly in recent years. While pip and setup.py were once the standard, newer tools offer more comprehensive solutions for managing dependencies and project configurations.
- Poetry: This tool provides a single pyproject.toml file for configuration, developer-friendly tooling for adding dependencies, and a lock file for consistent deployments .
- Pipenv: Similar to npm for Node.js or bundler for Ruby, Pipenv manages dependencies on a per-project basis. It creates a [Pipfile](https://packaging.python.org/en/latest/tutorials/managing-dependencies/) to track project dependencies .
- Hatch: This tool adheres strictly to Python PEP standards and offers innovative features like grouping dependencies and scripts into custom environments .
- PDM: Following current PEP standards, PDM provides good support for cross-platform lock files and includes a useful pdm export command to output a standard requirements.txt file .
- Rye: Developed by the creator of Flask, Rye has quickly gained popularity due to its opinionated approach to building Python packages .
Adopting pyproject.toml for Project Configuration
The pyproject.toml file has become a standard for configuring Python projects. It’s used not only by packaging tools but also by linters, type checkers, and other development tools .
Key sections in pyproject.toml include:
- [build-system]: Specifies the build backend and dependencies needed to build your project.
- [project]: Contains basic metadata like dependencies, name, and version.
- [tool]: Includes tool-specific configurations.
By adopting pyproject.toml, you’re standardizing your project configuration, making it easier to switch between different package managers if needed. This flexibility allows you to choose the tool that best suits your workflow, improving your overall development experience.
Harnessing the Power of New Python Syntax
Exploring Pattern Matching
Python 3.10 introduced structural pattern matching, a powerful feature that goes beyond a simple switch statement. This new syntax allows you to match and destructure complex data structures efficiently. With pattern matching, you can elegantly handle different cases in your code, making it more readable and maintainable .
To use pattern matching, you employ the match and case keywords. For example:
match command:
case "push":
print("pushing")
case "pull":
print("pulling")
case _:
print("Command not implemented")
This syntax enables you to match against various patterns, including sequences, mappings, and objects. You can even use wildcards and capture variables within patterns, making it a versatile tool for handling complex data structures .
Efficient Coding with the Walrus Operator
The walrus operator (:=), introduced in Python 3.8, allows you to assign values to variables within expressions. This operator can help you write more concise and efficient code. For instance, you can use it to simplify loop conditions or avoid redundant computations .
Here’s an example of how the walrus operator can streamline your code:
while (user_input := input("Enter command: ")) != "quit":
process_command(user_input)
This syntax combines the assignment and condition evaluation in one step, making your code more compact and potentially improving performance .
Enhancing Function Definitions with New Parameter Types
Python’s type hinting system has evolved to provide more precise ways to specify function parameters and return types. You can now use advanced type hints to make your code more self-documenting and easier to understand .
For example, you can use Callable to annotate functions:
from collections.abc import Callable
def process(func: Callable[[int], str]) -> None:
result = func(42)
print(result)
This annotation indicates that func should be a function that takes an integer and returns a string .
By leveraging these new Python syntax features, you can write more expressive, efficient, and maintainable code. Embrace these modern Python capabilities to enhance your development experience and produce higher-quality software.
Conclusion
To wrap up, mastering modern Python development has a significant impact on a programmer’s efficiency and code quality. The integration of type hinting, advanced project management tools, and new syntax features equips developers to create more reliable and maintainable software. These advancements not only streamline the coding process but also enhance collaboration among team members, leading to more robust and scalable applications.
As the Python ecosystem continues to evolve, staying up-to-date with these practices is crucial to remain competitive in the fast-paced tech world. By embracing these modern techniques, developers can unlock new possibilities in their projects and push the boundaries of what’s achievable with Python. The journey to become a skilled Python developer is ongoing, but these essential keys provide a strong foundation to build upon and excel in the ever-changing landscape of software development.
Article published on May 8, 2024
If you like this article, please share it: |
Tags: Development, Python, python development